Intelligent Reporting of Automated Test Execution
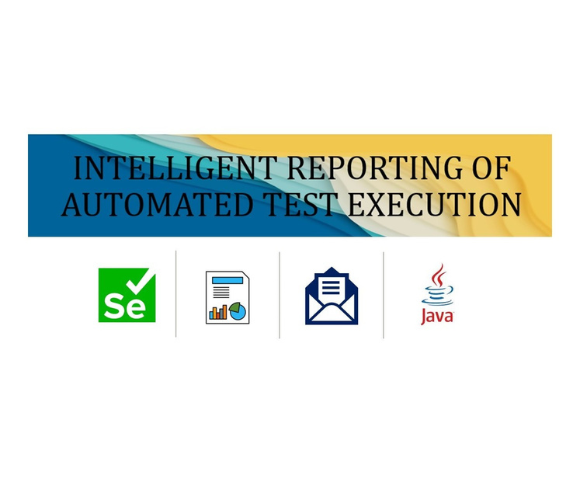
It is always important to share test execution results and log details after test execution. Doing this manually post every execution is time consuming and causes delays. Automating the email process with all test results details saves time, keeps everyone updated quickly and helps the team work together better.
Jenkins and other CI/CD tools can send email automatically using plugins. This article shows how to integrate email utility with selenium java test automation. This helps send emails automatically with test results, even when running tests on a local computer.
There are different ways to automate emails after Selenium Java tests:
- Email library
- JavaMail API
- Maven Plugins
- Third Party Services/API
We will focus on most commonly used two methods;
- Email library: Apache Commons Email is a robust library that simplifies email sending. It is a popular and user-friendly library that simplifies the process of sending email. It provides a high-level, easy-to-use API for sending emails via various protocols, including SMTP.
- JavaMail API: JavaMail API is used to send emails using SMTP server. JavaMail API supports both TLS and SSL authentication for sending emails. It provides classes and interfaces to send, receive, and manage email messages.
Let’s see how to implement in test automation framework,
- Apache commons Email Library:
- Add below dependency in pom.xml file
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-email</artifactId> <version>1.5</version> </dependency>
- Various instances of email classes can be created (HtmlEmail, SimpleEmail, and MultiPartEmail)
- Then set the necessary properties for your email, such as the sender’s email id, recipient’s email id, subject, and message content.
Here we are using HtmlEmail
import org.apache.commons.mail.DefaultAuthenticator; import org.apache.commons.mail.EmailAttachment; import org.apache.commons.mail.EmailException; import org.apache.commons.mail.HtmlEmail; import org.apache.commons.mail.MultiPartEmail; HtmlEmail email = new HtmlEmail(); email.setHostName("smtp.zoho.com"); email.setSmtpPort(587); email.setAuthenticator(new DefaultAuthenticator("sender@mail.com", "password")); email.setSSLOnConnect(true); email.addTo("receiver@mail.com"); email.setFrom("sender@mail.com "); email.setSubject("email Subject");
- Apache Commons Email allows to attach files and send HTML-formatted emails easily.
- send method used to send the email.
email.setHtmlMsg("<html><h1>Test Execution Report</h1></html>"); //Create the attachment EmailAttachment attachment = new EmailAttachment(); attachment.setPath(System.getProperty("user.dir") + "\src\test\resources\com.Reports\ZipFileToSend.zip"); attachment.setDisposition(EmailAttachment.ATTACHMENT); attachment.setDescription("set_description"); attachment.setName("name_to_the_file"); email.attach(attachment); //to Send email email.send();
2. JavaMail API:
- Add below dependency in pom.xml file
<dependency> <groupId>com.sun.mail</groupId> <artifactId>javax.mail</artifactId> <version>1.6.2</version> </dependency>
- Set up different properties such as email address, subject, reply to email, email body and attachment.
- SMTP server is used to send emails. JavaMail API supports both TLS (Transport Layer Security) and SSL (Secure Sockets Layer) authentication for sending emails.
- Replace SMTP Server and SMTP Port number with your actual details.
import java.util.Properties; import javax.mail.*; import javax.mail.internet.*; Properties props = new Properties(); props.put("mail.smtp.auth", "true"); props.put("mail.smtp.starttls.enable", "true"); props.put("mail.smtp.host", "SMTP Server"); props.put("mail.smtp.port", "SMTP Port number");
- Create a Session object using Session.getDefaultInstance(props, auth);.
- The Session will be used to create the email message and manage the email session.
- Provide valid sender’s email id as username and senders password in password.
Session session = Session.getInstance(props, new javax.mail.Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(username, password); } });
- Compose the email – Create a MimeMessage object to represent the email message and set the sender email id, recipient email id, email subject and body.
- Transport.send() method is used to send mail.
MimeMessage message = new MimeMessage(session); message.setFrom(new InternetAddress("sender@mail.com")); message.addRecipient(javax.mail.Message.RecipientType.TO, new InternetAddress("receiver@mail.com")); message.setSubject("Email Subject"); message.setText("Email Body"); Transport.send(message);
Test Automation Streamlining REST API Processes with Postman Automation Summary:In today's world, industries are increasingly focusing on the development of...
Uncategorized Enhancing Test Efficiency with Playwright, TestNG and Allure Summary:Playwright is an open-source library developed by Microsoft for automated browser...
Test Automation Continuous Integration and Delivery with Jenkins and GitHub Summary: Testing and deployment-related operations can be automated with Jenkins,...
Mobile App Test Automation with TDD Telecommunications (Cable) Location Performance Testing CONTEXT Elyments is an India-based mobile application similar to...